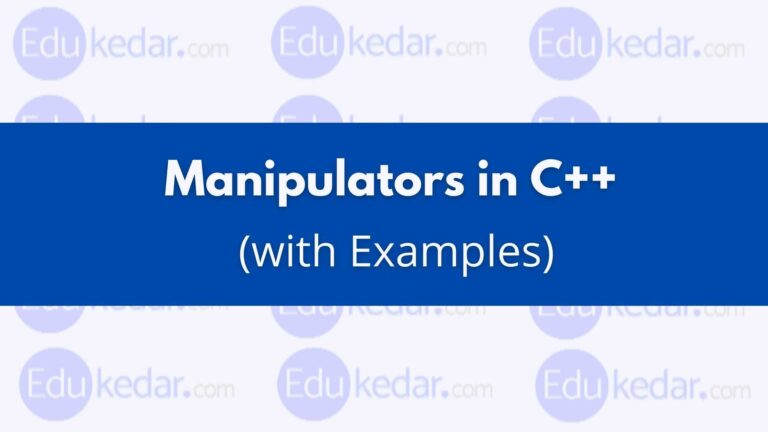
Manipulators are special functions that can be included in the I/O statement to alter the format parameters of a stream. To access manipulators, the file iomanip.h should be included in the program. In this article, we have shared the list of Manipulators in C++ and functions of manipulators with examples.
What are Manipulators in C++?
manipulators are simply an instruction to the output stream that modify the output in various ways. In other words, we can say that Manipulators are operators that are used to format the data display.
Advantages and Purpose of Manipulators
- It is mainly used to make up the program structure.
- Manipulators functions are special stream function that changes certain format and characteristics of the input and output.
- To carry out the operations of the manipulators <iomanip.h> must be included.
- Manipulators functions are specially designed to be used in conjunction with insertion (<<) and extraction (>>) operator on stream objects.
- Manipulators are used to changing the format of parameters on streams and to insert or extract certain special characters.
Types of Manipulators in C++
They are of two types one taking arguments and the other without argument.
1. Non-argument manipulators (Without Parameters)
Non-argument manipulators are also known as “Parameterized manipulators”. These manipulators require iomanip header. Examples are setprecision, setw and setfill.
2. Argumented manipulators (With parameters)
Argument manipulators are also known as “Non parameterized manipulators”. These manipulators require iostream header. Examples are endl, fixed, showpoint, left and flush.
Standard input/output Manipulators in C++
Here is the list of standard input/output Manipulators and their Functions in C++
- setw (int n) – To set field width to n
- Setbase – To set the base of the number system
- stprecision (int p) – The precision is fixed to p
- setfill (Char f) – To set the character to be filled
- setiosflags (long l) – Format flag is set to l
- resetiosflags (long l) – Removes the flags indicated by l
- endl – Gives a new line
- skipws – Omits white space in input
- noskipws – Does not omit white space in the input
- ends – Adds null character to close an output string
- flush – Flushes the buffer stream
- lock – Locks the file associated with the file handle
- ws – Omits the leading white spaces present before the first field
- hex, oct, dec – Displays the number in hexadecimal or octal or in decimal format
Must Read ➜ What is HTTP?
Manipulators in C++ With Examples
The manipulators in C++ are stream functions that change the properties of an input or output stream. It’s used to format the input and output streams by modifying the stream’s format flags and values.
The header file iomanip.h> contains a set of manipulator functions.
To utilize the manipulator functions in your program, you must include this header.
The following is a list of standard Functions in manipulator in C++.
The stream.h header file defines the hex, dec, oct, ws, endl, ends, and flush functions. The rest is defined in the header files iomanip.h.
► endl is a function in Manipulators in C++:
The endl character introduces a new line or a line feed. It is analogous to the “n” character in the C computer language, and C++ supports the old line feed.
As an example,
cout << "This line use line feed as example" << endl; cout << number3 << endl << number4 << endl;
You can use it anywhere and a new line character is added. It adds automatically.
Example Program #1
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std;o int main() { cout << " Hello World"; cout << endl; cout << " The Author is Damon salvatore"; cout << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
Hello World
The Author is Damon Salvatore
Must Read ➜ What is Cyclic Redundancy Check (CRC)?
► setbase() is a function in Manipulators in C++:
The setbase() manipulator is used to change the base of a number to a different value. The following base values are supported by the C++ language:
- hex (Hexadecimal = 16)
- oct (Octal = 8)
- dec (Decimal = 10)
Setbase () can change the base of a variable in addition to the base converters listed above. The manipulators hex, oct, and dec can change the basis of input and output numbers.
For example,
int number = 100; cout << "Hex Value =" << " " << hex << number << endl; cout << "Octal Value=" << " " << oct << number << endl; cout << "Setbase Value=" << " " << setbase(16) << number << endl;
The output of the above code is:
Hex Value = 0064
Octal Value = 144
Setbase Value= 0064
Example Program:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { //Variable Declaration int A,B,C; //Variable Initialization A = 2078; B = 3067; // Computing C C = A + B; // Printing Results cout << "A =" << dec << A <<endl; cout << "B =" << oct << B << endl; cout << "C = " << setbase(16) << C << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
A =2078
B =5773
C = 1419
► setw () is a function in Manipulators in C++:
The setw() function is an output manipulator that inserts whitespace between two variables. You must enter an integer value equal to the needed space.
setw ( int n)
As an example,
cout << number5 << number6 << endl;
cout << setw(2) << number5 << setw(5) << number6 << endl;
Example Program:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { //variable declaration int number1, number2, total; //variable initialization number1 = 100; number2 = 345; // expression total = number1 + number2; //printing output with setw cout << endl; cout << endl; cout << setw(5) << number1 << " + " << setw(5) << number2 << " = " << setw(6) << total << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
100 + 345 = 445
Must Read ➜ Recursion Function in Python
► setfill() is a function in Manipulators in C++:
It replaces setw(whitespaces )’s with a different character. It’s similar to setw() in that it manipulates output, but the only parameter required is a single character. It’s worth noting that a character is contained in single quotes.
setfill(char ch)
For example,
cout<< setfill('*') << endl; cout << setw(5) << number5 << setw(5) << number6 << endl;
The output of the above will be ‘*’ character between variable number5 and variable number6.
Example Program:
We will use the above setw() example with a little modifications.
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { //variable declaration int number1, number2, total; //variable initialization number1 = 100; number2 = 345; // expression total = number1 + number2; //printing output with setw cout << endl; cout << endl; cout << setfill('*') << endl; cout << setw(5) << number1 << " + " << setw(5) << number2 << " = " << setw(6) << total << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
**100 + **345 = ***445
► setprecision() is a function in Manipulators in C++:
It is an output manipulator that controls the number of digits to display after the decimal for a floating point integer. Make careful to include the ipmanip header in your program because the function is defined there.
As an example,
float A = 1.34255;
cout << setprecision(3) << A << endl;
The output is 1.34.
Example Program:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { //variable declaration float number1; //variable initialization number1 = 34.3358; //display the number using setprecision() cout << number1 << endl; cout << setprecision(2) << number1 << endl; cout << setprecision(3) << number1 << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
34.3358
34
34.3
► ends in C++
A string type value is given a null terminating character (‘0′) via the ends command. It doesn’t take an argument like endl; instead, it just adds a null.
Program as an example:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { // variable declaration int amount; // variable initialization amount = 333; //display as a string cout << " \" " << amount << ends << " \" " << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
” 333 “
Must Read ➜ What is Multiplexing?
► ws in C++
The ws is an input stream manipulator that removes all-white spaces, so if you have a string with multiple words, it will only display the first one and trim the rest.
Example Program:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { // variable declaration char name[125]; // read variable value cout << "Enter your name" << endl; cin >> ws; cin >> name; //display variable with whitespace cout << "The Name is "<< name << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
Enter your name
Damon
The Name is Damon
► flush in C++
The flush function removes all data from the output stream. It’s an output manipulator, so it won’t function with an input stream. The console screen’s output buffer is automatically emptied, however, the flush function is handy for cleaning the file output buffer following file operations. There are no arguments for this function.
Example Program:
#include <cstdlib> #include <iostream> #include <iomanip> using namespace std; int main() { //using Flush manipulator cout << "Hello" << endl; cout << "World" << endl; cout.flush(); cout << "Output Buffer Cleared" << endl; system("PAUSE"); return EXIT_SUCCESS; }
Output:
Hello
World
Output Buffer Cleared